Recently my router died and to get netflix flowing again, I went out and got the cheapest router I could find in the vicinity. I ended up with a D-Link DIR-842 on discount for 499 SEK. In the box, I discovered this:

It tells me to go to D-Link’s page for GPL licensed software to get the source code. It also lets me write a request the source code on physical media for a nominal fee for the media and handling. Something I naturally did (being an engineer on vacation).
While waiting for a reply, let’s have a look at the online version. When entering the URL provided you have to click through an agreement that I understand what GPL and LGPL means and that the files distributed comes with no warranties (they spend more words saying this – read it if you want the details). Clicking “I Agree” I get a popup (back to the 90’s) asking me to register my product to enjoy all the benefits of doing so. At the same time the main window continues to a list of all D-Link products containing (L)GPL software – very nice.
The list of products is 24 pages long, so I searched for my model name (DIR-842) and clicked the resulting link. This got me to the following table:
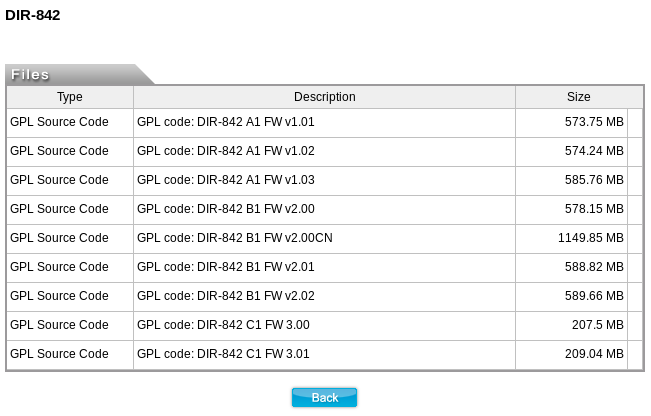
I wonder what separates firmware version 2.0CN (China?) from version 3.0. Having 950MB of translation tables seems odd, so something else must be the case. As I have firmware version 2.02 for hardware revision B1 I decided to download that bundle consisting of 590 MB of open source code as a tarball. At least, that was what I expected. Apparently, I don’t only get the sources, I also get a test report – great!
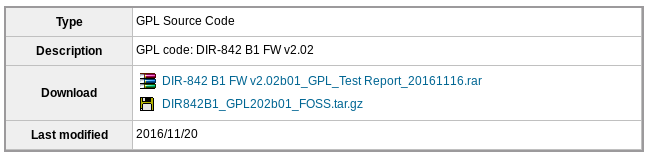
The test report rar file contained a pdf documenting the tests. On November 16, 2016, test engineer Mason Wu carried out the GPL SC tests consisting of the steps Firmware upgrade, Firmware downgrade, Compile the Open Source Software Licenses code, Check list (Before test Open Source Software Licenses), Open Source Software Digital Signature check, and License file check. All tests passed – time for celebrations!
To be completely honest, this report tells me nothing, as I don’t know what has been tested or what the test cases do. The only thing I learn is that I just put a device with almost two years old software on as my interface towards the Internet…
So what is in the source code tarball? You can see the directory structure of the tarball in the picture below (I guess someone named Lisa created the tarball). First of all, there are some licenses, then the source code.
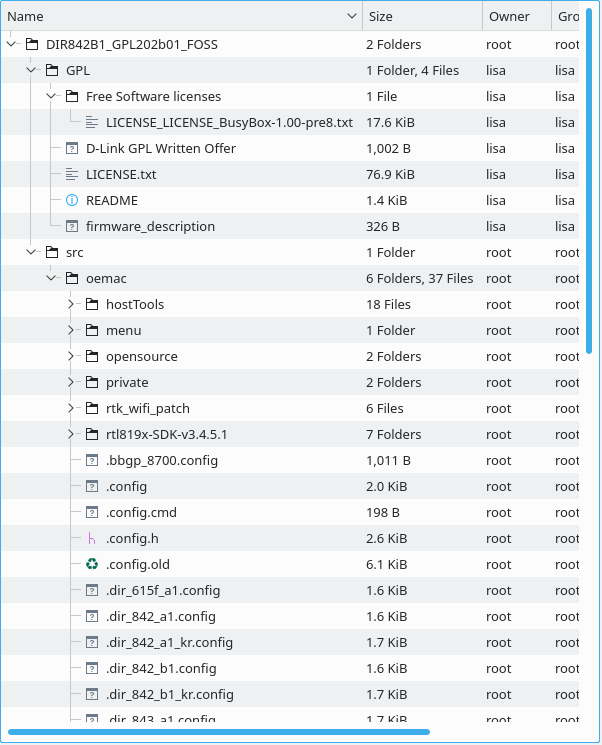
The source code is split into open source and private, where private is a set of prebuilt binaries, while open source comes with the whole source code. The open source software is licensed under the following licenses according to the LICENSE.txt:
- GNU General Public License Version 2 (GPLv2.0)
- GNU Lesser General Public License Version 2.1 (LGPLv2.1)
- BSD 2-clause license
- BSD 3-clause license
- Apache License 2.0
- zlib/libpng License
- MIT License v2.0
Looking into the realtek SDK (rtl819x-SDK-v3.4.5.1) I found the base Linux system (under rtl819x-SDK-v3.4.5.1/rtl819x). This is open source software found outside the open source directory.
There does not seem to be a license for the proprietary stuff. Not for the realtek related directories (rtk_wifi_patch and rtl819x-SDK-v3.4.5.1), nor the directory named private. I’m not sure what that means from a licensing perspective. I guess it is complicated. The nice thing with this is that I should be able to rebuild a new firmware image from this.
Continuing down the rabbit hole I’m getting really worried. Remember that this is an internet facing device. There are so many things I want to point out, but I’m on vacation so I can’t dig through the whole source code. Here are some snippets:
- The Makefiles outputs “It’s builded” when done. Kind of cute.
- Building is supported on CentOS 5.9 (32-bit version) with GCC 4.1.2 20080704 (Red Hat 4.1.2-54). This is a release from 17 January 2013.
- Building has to be done as root.
- For the proprietary stuff, there are .c.dep files showing what source files where used and their dependencies. Also, some headers are included without copyright information.
- The open source versions are really old. Some highlights:
- Samba 3.0.24 – from 2003. The CVE list for Samba is scary – this is a piece of software that should be updated.
- Kernel 2.6.30 – from 9 June 2009. End-of-lifed in October 2009).
However, the most critical issue is that code is not included in the release. Looking at the directory rtl819x-SDK-v3.4.5.1/rtl819x/toolchain there are a number of GCC tools (GPLv3 licensed, so the license list is incomplete) as well as binutils delivered only as binaries. These also include realtek confidental documents (see screenshot below).
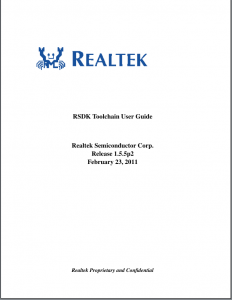
I’m stopping my dig here, but I will have to follow up my written request for the source code, unless the optical medium contains more. I thought they had learned…